How to Containerize Nest and Postgres using Docker
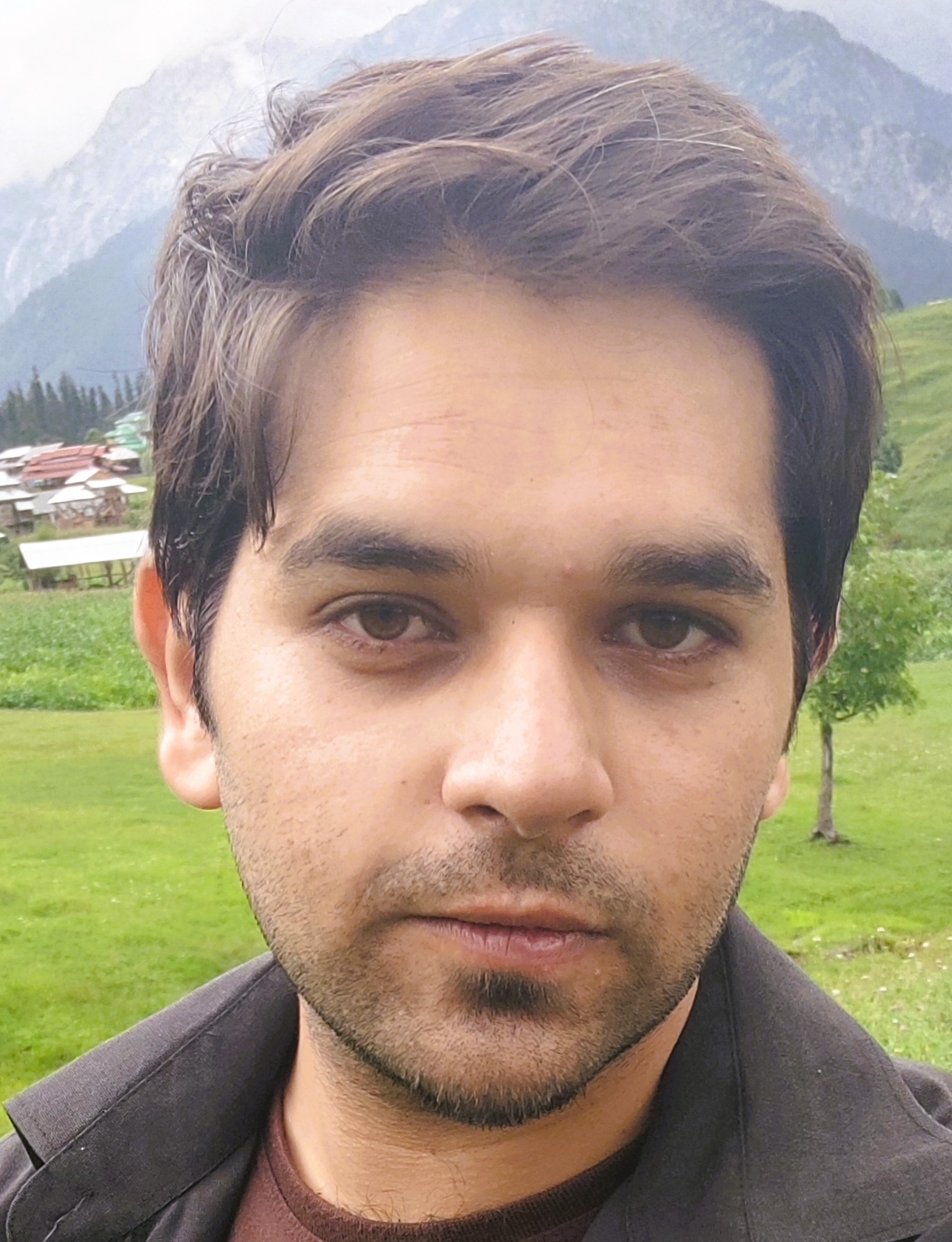
01-07-2024
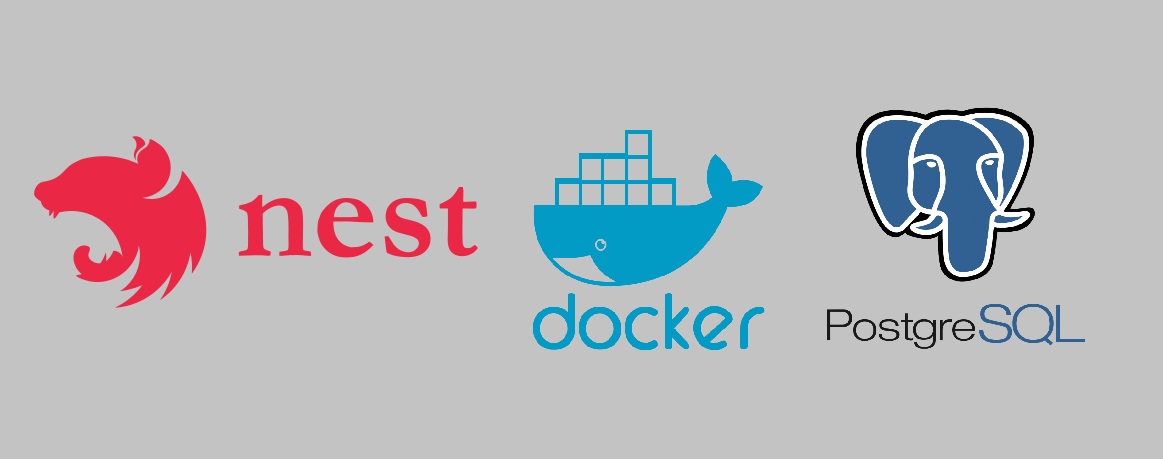
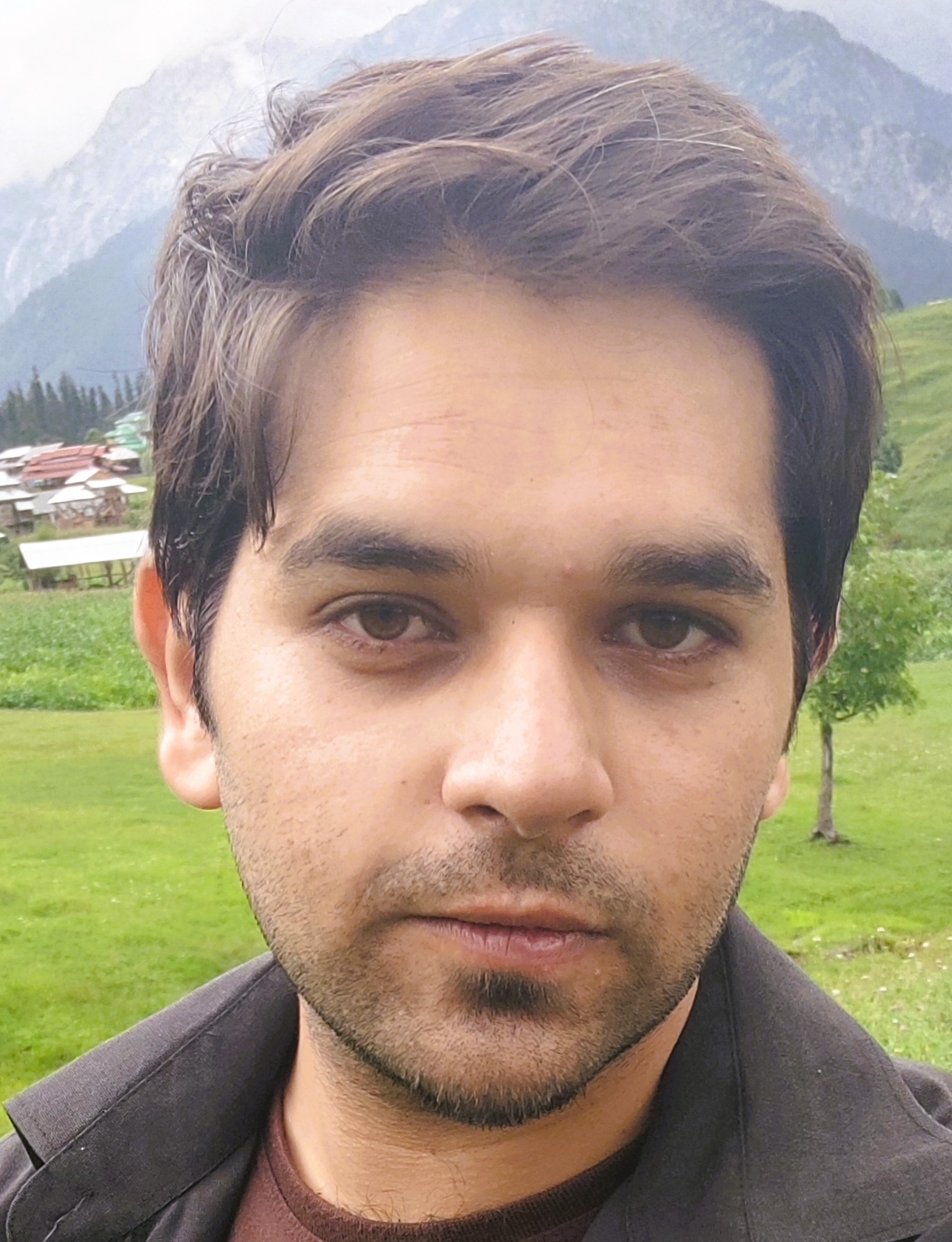
01-07-2024
In this post, I'll show you how to package your NestJS backend and PostgreSQL database into Docker containers.
Step 1: Install Docker Desktop
Ensure Docker Desktop is installed and running. This tool will manage Docker containers and images.
Step 2: Create a Dockerfile in your Nest project
Assuming you've already set up NestJS with TypeORM as described in Setting Up a NestJS Project with TypeORM, create a Dockerfile in your project's root directory.
FROM node:16
WORKDIR /backend/src/app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
EXPOSE 3000
CMD [ "npm", "run" , "start:prod" ]
Explanation of the Dockerfile:
FROM node:16
Base image with Node.js version 16.
WORKDIR /backend/src/app
Sets the working directory inside the container.
COPY package.json ./** Copies package files for dependency installation.
RUN npm install
Installs dependencies.
COPY . .
Copies the application code.
RUN npm run build
Builds the application.
EXPOSE 3000
Exposes port 3000.
CMD [ "npm", "run", "start" ]
Runs the production server.
Step 3: Create docker-compose.yml in the Nest root directory
Create a docker-compose.yml file to manage multiple Docker containers.
version: '3.9'
services:
nestapp:
container_name: nest-backend
image: backend:latest
build: .
ports:
- 3000:3000
environment:
- TYPE=${TYPE}
- HOST=${HOST}
- USERNAME=${USERNAME}
- PASSWORD=${PASSWORD}
- PORT=${PORT}
- DATABASE=${DATABASE}
depends_on:
- db
db:
container_name: db
image: postgres:12
ports:
- 5432:5432
environment:
- POSTGRES_USER=${POSTGRES_USER}
- POSTGRES_PASSWORD=${POSTGRES_PASSWORD}
- POSTGRES_DB=${POSTGRES_DB}
volumes:
- pgdata:/var/lib/postgresql/data
volumes:
pgdata: {}
Explanation of docker-compose.yml:
version: '3.9'
Docker Compose file format version.
Services
Defines nestapp and db services.
nestapp
NestJS backend service configuration.
container_name
Names the container nest-backend.
image
Uses the image backend:latest.
build
Builds the image from the current directory (.).
ports
Maps port 3000 of the container to port 3000 of the host.
environment
Sets environment variables for the application using values from the host system.
depends_on
Ensures nestapp starts after the db service.
db
PostgreSQL database service configuration.
container_name
Names the container db.
image
Uses the postgres:12 image.
ports
Maps port 5432 of the container to port 5432 of the host.
environment
Sets PostgreSQL environment variables using values from the host system.
volumes
Mounts a Docker volume pgdata to persist PostgreSQL data.
volumes
Defines a volume for PostgreSQL data persistence.
Step 4: Create .env file
Create an .env file in your project's root directory to store environment variables.
# For backend
TYPE=postgres
HOST=db
USERNAME=postgres
PASSWORD=mypassword
PORT=5432
DATABASE=mydatabase
# For db
POSTGRES_USER=postgres
POSTGRES_PASSWORD=mypassword
POSTGRES_DB=mydatabase
Step 5: Build and run Docker containers
Execute the following command to build and start Docker containers:
docker compose up
Conclusion
This guide will help you get started with Dockerizing your NestJS application alongside a PostgreSQL database, enabling you to manage your application environment more efficiently.